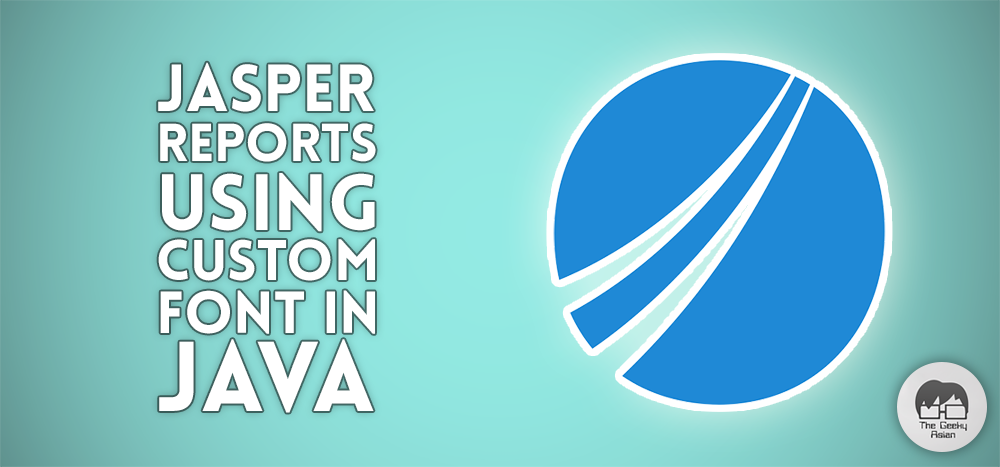
Sometimes its quite easy to find a library or a solution and implement it just by following a couple of steps. But sometimes it can be painful. Very painful.
That’s something I faced recently. I had to add custom fonts to jasper report in a Java project. Sadly the JasperReport community wasn’t too helpful as I couldn’t find an easier solution to my problem.
Many of the solutions asked me to create a jar package and then add it as a library in my java project. Uh, no thanks!
After being stuck for 3 days and nights, I was able to find a solution to this problem. An easier way!
Note that I am using Calibri font and its four versions. You can use use any font of your choice just by replacing the font files and configuring it on your own, following this tutorial.
Here is how it goes.
1. Maven Configuration
Let’s start by adding following two maven dependencies to our pom.xml file
a) JasperReports
The following dependency is an important one, that will add the JasperReport library in our Java project. With the help of this dependency we will be able to create our JasperReport.
<dependency> <groupId>net.sf.jasperreports</groupId> <artifactId>jasperreports</artifactId> <version>6.3.0</version> </dependency>
b) JasperReports Font
This dependency will allow you to configure custom fonts for your reports.
<dependency> <groupId>net.sf.jasperreports</groupId> <artifactId>jasperreports-fonts</artifactId> <version>6.0.0</version> </dependency>
2. Configuring Fonts
Once done with adding the dependency, create a directory named fonts in your resources directory. You can name this folder anything, but to be specific about it’s usage, I named it fonts.
. /src / main / resources / fonts
In the fonts directory, create a file named fonts.xml. This is a configuration file for the fonts. The file should look like this.
<?xml version="1.0" encoding="UTF-8"?> <fontFamilies> <fontFamily name="Calibri"> <normal><![CDATA[fonts/calibri/calibri.ttf]]></normal> <bold><![CDATA[fonts/calibri/calibri-bold.ttf]]></bold> <italic><![CDATA[fonts/calibri/calibri-italic.ttf]]></italic> <bolditalic><![CDATA[fonts/calibri/calibri-bold-Italic.ttf]]></bolditalic> <pdfEmbedded><![CDATA[true]]></pdfEmbedded> <exportFonts/> </fontFamily> </fontFamilies>
Right where the fonts.xml file is located, create a another directory named calibri and add your font files (.ttf) in the directory. As per the configuration xml, I have added following our font files in the calibri directory.
i. calibri.ttf (normal)
ii. calibri-bold.ttf (bold)
iii. calibri-italic.ttf (italic)
iv. calibri-bold-italic.ttf (bold-italic)
Here we are done with configuring font pack to be used in our JasperReports.
3. Jasper Report Properties
This is a final step of configuring fonts. In order to allow your Jasper Reports library to read fonts families, you need to to create a properties file named jasperreports_extension.properties in the resources directory
. /src / main / resources /
After creating your file, open it and add the following properties in your jasperreports_extension.properties file:
net.sf.jasperreports.extension.registry.factory.fonts=net.sf.jasperreports.engine.fonts.SimpleFontExtensionsRegistryFactory net.sf.jasperreports.extension.simple.font.families.myfontfamily=fonts/fonts.xml
4. Creating JRXML Template
Since we are done with the fonts configuration, its time to use the fonts in our JasperReport XML or JRXML Template. Lets create a .JRXML at the following directory path with the name jasperReport.jrxml
. /src / main / resources / reports
In my jasperReport.jrxml template, I have added four static text elements. Each static text element will be using each of our four font types.
Here is what my jasperReport.jrxml looks like.
<?xml version="1.0" encoding="UTF-8"?> <jasperReport xmlns="http://jasperreports.sourceforge.net/jasperreports" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://jasperreports.sourceforge.net/jasperreports http://jasperreports.sourceforge.net/xsd/jasperreport.xsd" name="CustomFont" pageWidth="595" pageHeight="200" columnWidth="555" leftMargin="20" rightMargin="20" topMargin="20" bottomMargin="20" uuid="0eb3df49-8342-4b94-9567-9afe83ee4297"> <property name="com.jaspersoft.studio.data.defaultdataadapter" value="One Empty Record"/> <queryString> <![CDATA[]]> </queryString> <background> <band splitType="Stretch"/> </background> <detail> <band height="125" splitType="Stretch"> <staticText> <reportElement x="127" y="30" width="100" height="30" uuid="a4cf64e9-d6c3-4ce9-9039-79800533550a"/> <textElement textAlignment="Left" verticalAlignment="Middle" markup="html"> <font fontName="Calibri" size="11.5"/> </textElement> <text><![CDATA[Calibri - Regular]]></text> </staticText> <staticText> <reportElement x="337" y="30" width="100" height="30" uuid="ea00d75c-4bc9-44cd-9385-45f9f5dacaf0"/> <textElement textAlignment="Left" verticalAlignment="Middle" markup="html"> <font fontName="Calibri" size="11.5" isBold="true"/> </textElement> <text><![CDATA[Calibri - Bold]]></text> </staticText> <staticText> <reportElement x="127" y="70" width="100" height="30" uuid="52ca47a2-6c40-4163-a3b2-26098001a2d4"/> <textElement textAlignment="Left" verticalAlignment="Middle" markup="html"> <font fontName="Calibri" size="11.5" isItalic="true"/> </textElement> <text><![CDATA[Calibri - Italic]]></text> </staticText> <staticText> <reportElement x="337" y="70" width="100" height="30" uuid="fb69e717-6483-4039-a012-4e3d6cd1404c"/> <textElement textAlignment="Left" verticalAlignment="Middle" markup="html"> <font fontName="Calibri" size="11.5" isBold="true" isItalic="true"/> </textElement> <text><![CDATA[Calibri - Bold Italic]]></text> </staticText> </band> </detail> </jasperReport>
If you have a look at the xml (or jrxml) template, you will see that I have used <font/> tag in each of the staticText elements. Here is how it works.
a. Normal
The first staticText element displays normal (or regular) calibri font. That is because I have used the following font element configuration.
<font fontName="Calibri">
b. Bold
Second staticText displays Calibri – Bold using the bold calibri font. It didn’t require me to use any complex science. I did that just by adding isBold flag and setting it true in the font element.
<font fontName="Calibri" isBold="true"/>
c. Italic
Third one displayed Calibri – Italic in an italic font format. That’s as easy as using the bold font. Just add isItalic flag in the font element, with true flag on it.
<font fontName="Calibri" size="11.5" isItalic="true"/>
d. Bold-Italic
Fourth one is a mix of Bold and Italic config and I would like you to make a guess on it. Well yes! All you need to do is add both, isBold and isItalic flags in the font element. Here is how it looks.
<font fontName="Calibri" size="11.5" isBold="true" isItalic="true"/>
With this, we have completed our report template configuration.
This is the final step. Lets create our pdf JasperReport!
We will create an App.java class with a static main method inside it. This class will initialize a JasperReport object and create a report.pdf file using our jasperReport.jrxml template as a source.
Here is how our App.java class would look like.
public class App { public static void main (String[] args){ String fileName = "report.pdf"; File file = new File(fileName); Resource resource = new ClassPathResource("reports/jasperReport.jrxml"); try (ByteArrayOutputStream bos = new ByteArrayOutputStream()) { JasperReport jasperReport = JasperCompileManager.compileReport(resource.getInputStream()); JasperPrint jasperPrint = JasperFillManager.fillReport(jasperReport, null, new JREmptyDataSource()); JRPdfExporter exporter = new JRPdfExporter(); exporter.setExporterInput(new SimpleExporterInput(jasperPrint)); SimpleOutputStreamExporterOutput simpleOutputStreamExporterOutput = new SimpleOutputStreamExporterOutput(bos); exporter.setExporterOutput(simpleOutputStreamExporterOutput); SimplePdfExporterConfiguration simplePdfExporterConfiguration = new SimplePdfExporterConfiguration(); simplePdfExporterConfiguration.setMetadataAuthor("TheGeekyAsian"); exporter.setConfiguration(simplePdfExporterConfiguration); exporter.exportReport(); FileUtils.writeByteArrayToFile(file, bos.toByteArray()); simpleOutputStreamExporterOutput.close(); } catch (Exception e){ e.printStackTrace(); } } }
Annnnndddd, done! All you have to do now is compile and execute App.java class. This will create a report.pdf file for you, using your custom fonts.
I have complied a complete code and put it on my GitHub repository in one place. You can find it here.
Feel free to drop your questions and feedback in the comments section below. Lets solve the problems together! Good luck reporting with JasperReports.
Thank you so much, this saved me so much time.
Note: something that is in the github repo that you didn’t mention inside the post was the need for the
jasperreports_extension.properties file so that the fonts.xml file is actually used
Thank you for pointing it out Henry!
I’ve updated the article and added the properties configuration part as well.
Cheers!
Thank you very much…. This helps alot. Keep up the good work Asian Geek.. All the very best.
Thanks a lot for this article. It helped me solved the error :
net.sf.jasperreports.engine.JRRuntimeException: Could not load the following font:
pdfFontName:
pdfEncoding: Cp1252
isPdfEmbedded : false
at net.sf.jasperreports.engine.export.JRPdfExporter.getFont(JRPdfExporter.java:2756)
I’m using jasperreports 6.14.0
That’s about nearly 3 years old post and still saving a lot of days of research for anyone struggling to incorporate a specific font into Jasper Reports and Java.
Very well put step by step and error free guide.
dude, you save me. i tried different way from other sources, none of them work. i try this way once, it works. Thank you so much. hope you always healthy.
mannnnnnn, thank you so much. i’ve been trying to generate a PDF with arabic text with different libraries for three days, yesterday I started with jasper but till now I didn’t manage to register the fontI need. thank you so much for this post!
I Salute you my friend
My bro…
I tried several days with many solutions that I found on the internet, but yours is undoubtedly the only one that worked for me and the only one in which the user explains his idea well.
Thank you so much.
Thanks a lot THEGEEKYASIAN!!!!. You made my work easy. I faced a lot of issues in my Jasper Report project where i have used the font Trebuchet MS. Your solution worked like magic!!!. Thanks again and Cheers!.